Generating a Private AES256-CBC Key
- Openssl Generate Aes 256 Key
- C 2b 2b Openssl Generate Aes 256 Key Size
- Openssl Generate Aes 128 Key
- Openssl Create Aes 256 Key
1. We are going to create a private key for your website or server. Make sure you have OpenSSL installed in your server before we commence. Type the following into the terminal.
# openssl genrsa -aes256 -out domain.key 4096
or

IMPORTANT - ensure you use a key. In this example we are using 256 bit AES (i.e. The./ if (1!= EVPDecryptInitex (ctx, EVPaes256ecb , NULL, key)) handleErrors ; /. Provide the message to be decrypted, and obtain the plaintext output. Jun 23, 2012 There is also the option to use the EVPBytesToKey function which is a PBKDF. This function, as I called it, will generate a 256 bit key in CBC mode, with a salt and passphrase that are random data (the password being random data is just for demonstration purposes).
# openssl genrsa -aes256 -out domain.key 2048
Enter pass phrase for domain.key
Note: Write this down and make sure you keep it in a safe place or memorize it.
2. Once you have typed your password, it will generate your private key.
Generating a CSR Request for SSL Certificate
3. To generate a CSR Request we type the following to generate a request from the private key.
# openssl req -out domain.csr -key domain.key -new
4. Enter the passphrase that you created for the key
5. Enter Country Name (2 letter code) [XX] US
6. Enter State or Province Name (full name) Michigan
7. Locality Name (eg, city) [Default City] 'City Where You Live'
8. Organization Name: Company Name
9. Organizational Unit Name
10. Common Name (eg, your name or your server's hostname)
11. Email Address
12. Challange Password

13. An optional company name
15. Then it will generate the CRS File. Then you submit it.
What is Special about CBC?
CBC stands for cipher-block chaining. Its a mode of operation for a block cipher (one in which a sequence of bits are encrypted as a single unit or block with a cipher key applied to the entire block).
AES encryption and decryption program in C
The thing about encryption is not the actual functions, but the flow and what to do with the information as you encrypt and decrypt. That is what makes this type of work hard, not just the fact you can encrypt things.
Here is the simple “How to do AES-128 bit CBC mode encryption in c programming code with OpenSSL” First you need to download standard cryptography library called OpenSSL to perform robust AES(Advanced Encryption Standard) encryption, But before that i will tell you to take a look at simple C code for AES encryption and decryption, so that you are familiar with AES cryptography APIs which
Example: C program to encrypt and decrypt the string using Caesar Cypher Algorithm. For encryption and decryption, we have used 3 as a key value. While encrypting the given string, 3 is added to the ASCII value of the characters.
Implement encryption and decryption with OpenSSL
Tutorial: AES Encryption and Decryption with OpenSSL , Encrypting: OpenSSL Command Line. To encrypt a plaintext using AES with OpenSSL, the enc command is used. The following command will OpenSSL is a powerful cryptography toolkit that can be used for encryption of files and messages. If you want to use the same password for both encryption of plaintext and decryption of ciphertext, then you have to use a method that is known as symmetric-key algorithm.
EVP Symmetric Encryption and Decryption, When used in combination with -d openssl will first base64 decode the input and then decrypt it. -p -k world - This is the same as our encryption example, -p is printing the various inputs to the decryption algorithm and -k world is specifying the passphrase to use for decryption. OpenSSL is specifically concerned with implementing SSL and TLS which are protocols for encrypting data over a network. Since you are just looking to encrypt a file, it is possible to use OpenSSL but not ideal. Instead, I would use something like BeeCrypt or Crypto++® Library 5.6.0 which both provide examples for their use.
The Developer Toolbox: Encryption/Decryption with the OpenSSL , Check out this link it has a example code to encrypt/decrypt data using then look in the implementation of EVP and aeh api implementation. Simple text encryption/decryption with openssl. GitHub Gist: instantly share code, notes, and snippets.
AES file encryption in C
The code you compiled is probably just a library, exporting functions such as aes_encrypt().. To be able to compile it into an executable, you need to tell the library to actually do something, like here (just to get the idea; that code probably uses a different library).
If you are just after AES and do not mind losing flexibility (i.e. you will not replace it with another cryptographic algorithm at some time) then Brian Gladman's AES implementation is a popular choice (both for performance and portability). This is the kind of code which you embed in your own source code.
Here i use AES-128 bit CBC mode Encryption, where 128 bit is AES key length. We can also use 192 and 256 bit AES key for encryption in which size and length of key is increased with minor modification in following code. Compiling and Installing OpenSSL Before compiling this code, you need OpenSSL library which you can download from here
Openssl AES encryption example in C
I am trying to write a sample program to do AES encryption using Openssl. This answer is kind of popular, so I'm going to offer something more up-to-date since OpenSSL added some modes of operation that will probably help you. First, don't use AES_encrypt and AES_decrypt. They are low level and harder to use.
AES Encryption -Key Generation with OpenSSL (Get Random Bytes for Key) [stackoverflow.com] How to do encryption using AES in Openssl [stackoverflow.com] AES CBC encrypt/decrypt only decrypts the first 16 bytes [stackoverflow.com] Initialization Vector [wikipedia.org] AES encryption/decryption demo program using OpenSSL EVP apis [saju.net.in]
Here is the simple “How to do AES-128 bit CBC mode encryption in c programming code with OpenSSL” First you need to download standard cryptography library called OpenSSL to perform robust AES(Advanced Encryption Standard) encryption, But before that i will tell you to take a look at simple C code for AES encryption and decryption, so that you are familiar with AES cryptography APIs which
AES encryption and decryption in C++
A simple example of using AES encryption in Java and C. · GitHub, buffer = calloc(1, buffer_len);. strncpy(buffer, plaintext, buffer_len);. printf('Cn');. printf('plain: %sn', plaintext);. encrypt(buffer, buffer_len, IV, key, keysize);. The thing about encryption is not the actual functions, but the flow and what to do with the information as you encrypt and decrypt. That is what makes this type of work hard, not just the fact you can encrypt things.
C - Encrypt and decrypt a string with AES, As @Morten Jensen suggested, you can use the CTR-mode IE: AES_CTR_xcrypt_buffer int main() { uint8_t key[] = 'secret key 123'; uint8_t in[] Here is the simple “How to do AES-128 bit CBC mode encryption in c programming code with OpenSSL” First you need to download standard cryptography library called OpenSSL to perform robust AES(Advanced Encryption Standard) encryption, But before that i will tell you to take a look at simple C code for AES encryption and decryption, so that you are familiar with AES cryptography APIs which
How to implement AES encryption in C?, I know this is a bit of a late answer. If you're still wondering, you just need to copy aes.h from the library you got the aes.c-code from. You then save it in the same Whenever the word encryption comes to our mind, we will move to the topic AES (Advanced Encryption Standard). But today I came up with an ideology of using Public Key Cryptography. One can perform encryption and decryption by the source code provided below but to better understand the concept, please read the theory.
AES encryption and decryption in C++ using OpenSSL
Openssl Generate Aes 256 Key

You should not use AES_encrypt and friends. You should be using EVP_* functions. See EVP Symmetric Encryption and Decryption on the OpenSSL wiki. In fact, you should probably be using authenticated encryption because it provides both confidentiality and authenticity.
Here i use AES-128 bit CBC mode Encryption, where 128 bit is AES key length. We can also use 192 and 256 bit AES key for encryption in which size and length of key is increased with minor modification in following code. Compiling and Installing OpenSSL Before compiling this code, you need OpenSSL library which you can download from here
AES encryption/decryption demo program using OpenSSL EVP apis That’s a lot of information to process, can I just have a quick demo to see how it’s working? Sure,
Open ssl aes 128
Encrypt files using AES with OPENSSL | by Kekayan, mode aes[128|192|256] Alias for aes-[128|192|256]-cbc aes-[128|192|256]-cfb 128/192/256 bit AES in 128 bit CFB mode aes-[128|192|256]-cfb1 128/192/256 # openssl genrsa -aes128 -out key.pem This command uses AES 128 only to protect the RSA key pair with a passphrase, just in case an unauthorized person can get the key file. When your Apache server starts up, it must decrypt the key in memory to use it.
Enc, The cryptographic keys used for AES are usually fixed-length (for example, 128 or 256bit keys). Because humans cannot easily remember long use openssl to realize aes128 encrypt/decrypt string with c++11 and provide .so to be called by others - zzg228/AES128
/docs/man1.1.0/man1/openssl-enc.html, #include <openssl/evp.h> #include <openssl/aes.h> #include A 128 bit IV */ static const unsigned char iv[] = '0123456789012345'; Here i use AES-128 bit CBC mode Encryption, where 128 bit is AES key length. We can also use 192 and 256 bit AES key for encryption in which size and length of key is increased with minor modification in following code. Compiling and Installing OpenSSL Before compiling this code, you need OpenSSL library which you can download from here
Java openssl AES encryption
Java equivalent of an OpenSSL AES CBC encryption, Following is a Java program to decrypt the above OPENSSL encryption (it requires Java 8): import java.nio.charset.StandardCharsets; import Following is a Java program to decrypt the above OPENSSL encryption (it requires Java 8): 32508961/java-equivalent-of-an-openssl-aes-cbc-encryption for what looks
Mine of Information - Symmetric Encryption in Java, BouncyCastleProvider; /** * Implement code to decrypt a file that has been encrypted by openssl using the aes-***-cbc algorithm and a <i>password</i>. A more secure encryption algorithm is AES – Advanced Encryption Standard which is a symmetric encryption algorithm. AES encryption is used by U.S. for securing sensitive but unclassified material, so we can say it is enough secure. Read More : Java AES 256 Encryption Decryption Example 1.
minibackup/OpensslAES.java at master · chmduquesne/minibackup , import javax.crypto.spec.SecretKeySpec;. public class OpensslAES {. /*. * Derive an key and IV from a password and a salt. * using OpenSSL's non-standard The Advanced Encryption Standard (AES, Rijndael) is a block cipher encryption and decryption algorithm, the most used encryption algorithm in the worldwide. The AES processes block of 128 bits using a secret key of 128, 192, or 256 bits. This article shows you a few of Java AES encryption and decryption examples:
AES 256 C++ example
kokke/tiny-AES-c: Small portable AES128/192/256 in C, Small portable AES128/192/256 in C. Contribute to kokke/tiny-AES-c development 2001 ED Appendix F: Example Vectors for Modes of Operation of the AES. Congratulations, you just decoded encrypted text using AES-256 encryption! CommonCrypto’s CCCrypt C function looks very scary but it’s actually really easy to use. Most of the code in the category involves getting the raw bits from the hex strings and putting them into C byte arrays. As we’re using C functions we have to calculate how
A simple example of using AES encryption in Java and C. · GitHub, A simple example of using AES encryption in Java and C. - AES.c. Cross Platform AES 256 GCM Encryption and Decryption (C++, C# and Java) Introduction While working in security, identity management and data protection fields for a while, I found a very few working examples in the public domain on cross platform encryption based on AES 256 GCM algorithm.
How to implement AES encryption in C?, I know this is a bit of a late answer. If you're still wondering, you just need to copy aes.h from the library you got the aes.c-code from. You then save it in the same The first example below will illustrate a simple password-based AES encryption (PBKDF2 + AES-CTR) without message authentication (unauthenticated encryption). The next example will add message authentication (using the AES-GCM mode), then will add password to key derivation (AES-256-GCM + Scrypt).
Error processing SSI fileOpenssl generate aes-256 key base64
Generating secure random strong encryption keys • NServiceBus , Generating secure random strong encryption keys. There are multiple ways of generating an encryption key. openssl rand -base64 32 The command I'm using to generate the key is: $ openssl enc -aes-256-cbc -k secret -P -md sha1 salt=E2EE3D7072F8AAF4 key
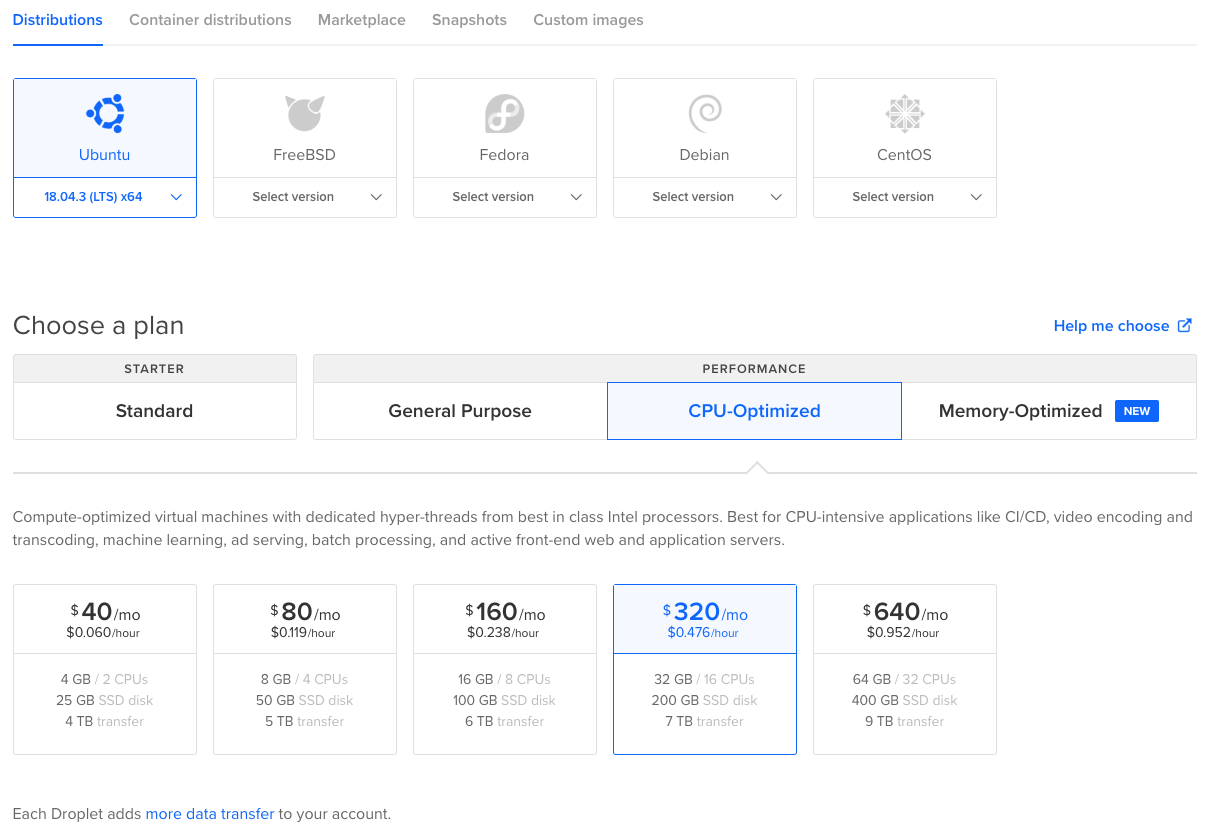
Using AES with OpenSSL to Encrypt Files, Generate an AES key plus Initialization vector (iv) with openssl and -aes-256-cbc -in message.txt -out message.txt.enc -base64 -K <key> -iv In your case the SecureRandom.hex is returning base64 encoded bits, while OpenSSL::Cipher::Cipher.new('aes-256-gcm').random_key is returning ASCII encoded bits (where bit sequences that have no ASCII representation are prefixed with x and hex encoded).
Why is OpenSSL generated 256-bit AES key 64 characters in length , I thought I could just read the key string and base64 decode it to get a 256-bit AES key, but that didn't work because 64 characters turned into a Generate an AES key plus Initialization vector (iv) with openssl and; how to encode/decode a file with the generated key/iv pair; Note: AES is a symmetric-key algorithm which means it uses the same key during encryption/decryption. Generating key/iv pair. We want to generate a 256-bit key and use Cipher Block Chaining (CBC).
Error processing SSI fileC 2b 2b Openssl Generate Aes 256 Key Size
Openssl/aes h
openssl/aes.h at master · openssl/openssl · GitHub, TLS/SSL and crypto library. Contribute to openssl/openssl development by creating an account on GitHub. # ifndef OPENSSL_AES_H # define OPENSSL_AES_H # pragma once # include < openssl/macros.h > # ifndef OPENSSL_NO_DEPRECATED_3_0 # define HEADER_AES_H # endif # include < openssl/opensslconf.h > # include < stddef.h > # ifdef __cplusplus: extern ' C ' {# endif # define AES_BLOCK_SIZE 16 # ifndef OPENSSL_NO_DEPRECATED_3_0 # define AES_ENCRYPT 1
OpenSSL: include/openssl/aes.h File Reference, Functions. const char *, AES_options (void). int, AES_set_encrypt_key (const unsigned char *userKey, const int bits, AES_KEY *key). int, AES_set_decrypt_key 1 /* crypto/aes/aes.h -*- mode:C; c-file-style: 'eay' -*- */ 2 /* 3 * Copyright (c) 1998-2002 The OpenSSL Project.
OpenSSL: include/openssl/aes.h Source File, 1 /* crypto/aes/aes.h -*- mode:C; c-file-style: 'eay' -*- */ 19 * 'This product includes software developed by the OpenSSL Project. 20 * for use in the OpenSSL Alternately, instead of the two sed's, you can open arm_arch.h, copy the defines and paste them directly into aes-armv4.S. Take care when using arm_arch.has it carries the OpenSSL license. After the two fixups aes-armv4.Sis ready to be compiled by GCC.
Error processing SSI fileAES_cbc_encrypt example
Openssl Generate Aes 128 Key
How do I use AES_CBC_Encrypt 128 openssl properly in Ubuntu , your whole example won't work with sha1. MD5_DIGEST_LENGTH and has 16 bytes (128 bit) just by coincidence. The initialization vector also C++ (Cpp) AES_cbc_encrypt - 30 examples found. These are the top rated real world C++ (Cpp) examples of AES_cbc_encrypt extracted from open source projects. You can rate examples to help us improve the quality of examples.
C++ (Cpp) AES_cbc_encrypt Examples, C++ (Cpp) AES_cbc_encrypt - 30 examples found. These are the top rated real world C++ (Cpp) examples of AES_cbc_encrypt extracted from open source This examples assumes you've filled the variable named key with the 32 bytes of the AES key (see How to generate an AES key), iv with 16 bytes of random data for use as the Initialization Vector (IV) and input with 40 bytes of input data, and zeroized the rest of input. The CBC mode for AES assumes that you provide data in blocks of 16 bytes
Openssl Create Aes 256 Key
aes_cbc function, The key is a raw vector, for example a hash of some secret. When no shared secret is aes_cbc_encrypt(data, key, iv = rand_bytes(16)). aes_cbc_decrypt(data Encrypt Decrypt message using AES-128 CBC in java (with example). We will generate random string and encrypt/decrypt the generated message using AES CBC.
Error processing SSI file